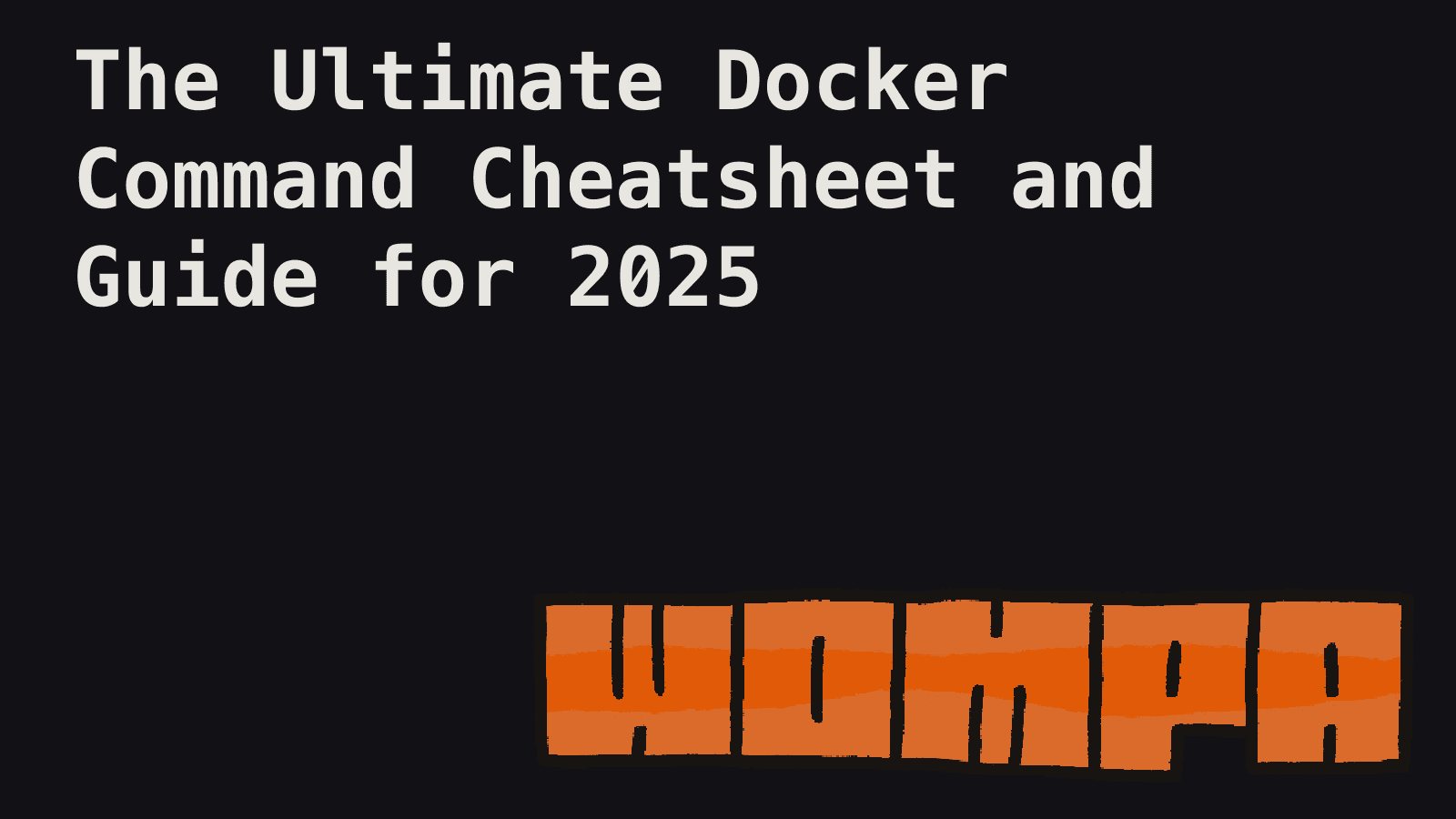
In the rapidly evolving landscape of software development and deployment, Docker has emerged as a revolutionary technology. As we approach 2025, the significance of containerization in modern software architecture is more pronounced than ever. This comprehensive guide is designed to equip both seasoned DevOps engineers and curious developers with the knowledge and tools to master Docker.
Introduction: The Power of Containerization
Docker has revolutionized the way we develop, ship, and run applications. By encapsulating software in containers, Docker ensures that applications work seamlessly across different environments, from a developer's laptop to a production server. This consistency eliminates the age-old problem of "it works on my machine" and streamlines the entire software development lifecycle.
As we look towards 2025, the adoption of Docker and containerization continues to grow. Organizations of all sizes are leveraging Docker to improve their development workflows, enhance scalability, and reduce operational overhead. Whether you're building microservices, deploying machine learning models, or managing complex web applications, Docker provides the tools and flexibility to meet your needs.
Getting Started with Docker: Installation and Setup
Before diving into commands, let's ensure you have Docker properly installed and configured.
Installation
To install Docker on Linux:
curl -fsSL https://get.docker.com -o get-docker.sh && sudo sh get-docker.sh
For other operating systems, visit the official Docker documentation.
Verifying the Installation
After installation, verify Docker is working correctly:
docker --version
docker run hello-world
Docker Hub Authentication
To access and push images to Docker Hub:
docker login
docker logout # When you're done
Essential Docker Commands: Container Lifecycle Management
Running Containers
Docker allows you to run containers with various configurations, including port mapping and resource limits:
# Run a container in detached mode
docker run -d --name mycontainer -p 8080:80 nginx
# Run a container interactively
docker run -it ubuntu /bin/bash
# Run a container with resource limits
docker run -d --name mycontainer --cpus 0.5 --memory 512m nginx
Listing Containers
To view and manage your running and stopped containers:
# List running containers
docker ps
# List all containers (including stopped)
docker ps -a
# List container sizes
docker ps -s
Starting, Stopping, and Restarting Containers
Control the state of your containers with these commands:
# Start a stopped container
docker start mycontainer
# Stop a running container
docker stop mycontainer
# Restart a container
docker restart mycontainer
# Pause a running container
docker pause mycontainer
# Unpause a paused container
docker unpause mycontainer
# Kill a running container
docker kill mycontainer
Removing Containers
Clean up unused containers and manage your Docker environment:
# Remove a stopped container
docker rm mycontainer
# Force remove a running container
docker rm -f mycontainer
# Remove all stopped containers
docker container prune
# Remove all containers (running and stopped)
docker rm -f $(docker ps -aq)
Executing Commands in Containers
Interact with running containers and manage files:
# Execute a command in a running container
docker exec -it mycontainer /bin/bash
# Execute a command as root in a running container
docker exec -it -u root mycontainer /bin/bash
# Copy files between host and container
docker cp /host/path/file.txt mycontainer:/container/path/
docker cp mycontainer:/container/path/file.txt /host/path/
Viewing Container Logs and Information
Monitor and debug your containers with these commands:
# View container logs
docker logs mycontainer
# Follow container logs
docker logs -f mycontainer
# Show the last N lines of container logs
docker logs --tail <number> mycontainer
# Show container resource usage statistics
docker stats mycontainer
# Display the running processes of a container
docker top mycontainer
# Display detailed information about a container
docker inspect mycontainer
# Show changes to files or directories on a container's filesystem
docker diff mycontainer
Image Management: Building, Tagging, and Pushing
Listing and Pulling Images
Manage your local image repository and fetch images from Docker Hub:
# List local images
docker images
# Pull an image from Docker Hub
docker pull nginx
Building Images
Create Docker images from your application code:
# Build an image from a Dockerfile
docker build -t myimage:latest .
# Build with a specific Dockerfile
docker build -f Dockerfile.dev -t myimage:dev .
# Build without using cache
docker build --no-cache .
Tagging Images
Version and organize your images:
# Tag an image
docker tag myimage:latest myrepo/myimage:v1.0
Pushing Images to a Registry
Share your images with others or deploy to remote environments:
# Push an image to Docker Hub
docker push myrepo/myimage:v1.0
Removing Images
Manage disk space by removing unnecessary images:
# Remove an image
docker rmi myimage:latest
# Remove all unused images
docker image prune -a
# Remove all images
docker rmi $(docker images -q)
Inspecting Images
Get detailed information about your images:
# Inspect an image
docker image inspect myimage:latest
# Show the history of an image
docker history myimage:latest
Saving and Loading Images
Transfer images between systems without using a registry:
# Save an image to a tar archive
docker save myimage:latest > myimage.tar
# Load an image from a tar archive
docker load < myimage.tar
Networking in Docker: Connecting Containers
Listing and Creating Networks
Manage Docker networks for container communication:
# List networks
docker network ls
# Create a network
docker network create mynetwork
Connecting and Disconnecting Containers
Manage container network connections:
# Connect a container to a network
docker network connect mynetwork mycontainer
# Disconnect a container from a network
docker network disconnect mynetwork mycontainer
Inspecting Networks
Get detailed information about a Docker network:
# Inspect a network
docker network inspect mynetwork
Removing Networks
Clean up unused networks:
# Remove a network
docker network rm mynetwork
Volume Management: Persistent Data Storage
Listing and Creating Volumes
Manage Docker volumes for persistent data storage:
# List volumes
docker volume ls
# Create a volume
docker volume create myvolume
Using Volumes with Containers
Demonstrate how to use a volume with a container:
# Run a container with a volume
docker run -d --name mycontainer -v myvolume:/app/data nginx
Inspecting Volumes
Get detailed information about a volume:
# Inspect a volume
docker volume inspect myvolume
Removing Volumes
Clean up unused volumes:
# Remove a volume
docker volume rm myvolume
# Remove all unused volumes
docker volume prune
Docker Compose: Managing Multi-Container Applications
Docker Compose simplifies the management of multi-container applications:
# Start services defined in docker-compose.yml
docker-compose up -d
# Stop services defined in docker-compose.yml
docker-compose down
# View logs of services
docker-compose logs
# Scale a service
docker-compose up -d --scale web=3
# Rebuild services
docker-compose build
# List containers managed by Compose
docker-compose ps
# Run a command in a service container
docker-compose exec <service_name> <command>
Docker Swarm: Container Orchestration
Docker Swarm provides native clustering and orchestration capabilities:
# Initialize a swarm
docker swarm init
# Join a swarm as a worker
docker swarm join --token <worker-token> <manager-ip>:<port>
# List nodes in the swarm
docker node ls
# Deploy a stack to the swarm
docker stack deploy -c docker-compose.yml mystack
# List services in the stack
docker stack services mystack
# Remove a stack
docker stack rm mystack
# Leave the swarm
docker swarm leave --force
Resource Management and Monitoring
Monitor and manage resource usage in your Docker environment:
# View Docker disk usage
docker system df
# View detailed container resource usage
docker stats
# Update a container's resource limits
docker update --memory <limit> <container_name>
Cleanup and Pruning
Maintain a healthy Docker environment with regular cleanup:
# Remove all unused containers, networks, images, and volumes
docker system prune -a
# Remove all stopped containers
docker container prune
# Remove all unused images
docker image prune -a
# Remove all unused volumes
docker volume prune
# Remove all unused networks
docker network prune
Security and Scanning
Identify and address security vulnerabilities in your Docker images:
# Scan an image for vulnerabilities
docker scan myimage:latest
# View image vulnerability details
docker scout cves myimage:latest
# Compare two images for vulnerabilities
docker scout compare --to myimage:latest myimage:v2
Advanced Docker Techniques
Multi-Stage Builds
Create smaller, more efficient images with multi-stage builds:
# Build stage
FROM node:14 AS build
WORKDIR /app
COPY package*.json ./
RUN npm install
COPY . .
RUN npm run build
# Production stage
FROM nginx:alpine
COPY --from=build /app/dist /usr/share/nginx/html
EXPOSE 80
CMD ["nginx", "-g", "daemon off;"]
Cross-Platform Builds
Build images for multiple architectures:
docker buildx build --platform linux/amd64,linux/arm64 -t myapp:latest .
Health Checks
Implement health checks in your Dockerfile to ensure your containers are running correctly:
HEALTHCHECK --interval=30s --timeout=3s \
CMD curl -f http://localhost/ || exit 1
Using Docker as a Development Environment
Create a disposable development environment:
docker run -it --rm -v $(pwd):/app -w /app node:14 bash
Troubleshooting and Debugging
Invaluable commands for troubleshooting Docker-related issues:
# View Docker events in real-time
docker events
# Get container exit code
docker wait <container>
# Run a network troubleshooting toolkit
docker run --net=host --privileged -it nicolaka/netshoot
# Debug a container's filesystem
docker export <container> > container.tar
docker import container.tar
# Run Docker daemon in debug mode
dockerd --debug
Docker Best Practices
- Use official base images
- Minimize the number of layers in your Dockerfile
- Use multi-stage builds to reduce image size
- Implement proper tagging strategies
- Regularly update and patch your images
- Use Docker Compose for multi-container applications
- Implement logging and monitoring solutions
- Regularly perform security scans on your images
- Use volumes for persistent data
- Implement resource limits on your containers
Conclusion: Embracing the Future of Containerization
As we look towards 2025 and beyond, Docker continues to be a cornerstone of modern software development and deployment. By mastering the commands and techniques outlined in this guide, you'll be well-equipped to leverage the full power of containerization in your projects.
Remember, the key to success with Docker lies in continuous learning and experimentation. As the ecosystem evolves, stay curious and keep exploring new ways to optimize your containerized applications.
Whether you're building microservices, deploying machine learning models, or managing complex web applications, Docker provides the flexibility and tools to meet your needs. Embrace the power of containerization, and unlock new levels of efficiency and scalability in your software development journey.
Happy Dockerizing!